译(三十二)-Python删除字典元素
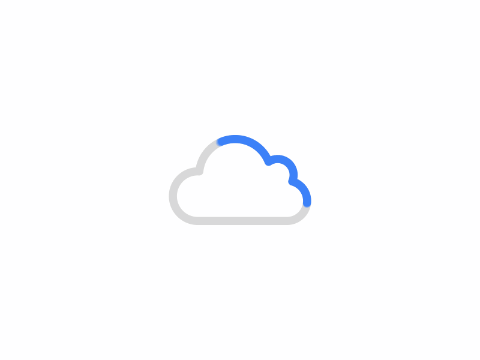
如有翻译问题欢迎评论指出,谢谢。
删除字典元素
richzilla asked:
- 如何在Python的字典中删除一个元素?
- 此外,如果我希望获得一个修改后的新字典,并且原字典还是未修改的样子,应该怎么办?
Answers:
Greg Hewgill - vote: 2142
del
语句可以删除元素:del d[key]
但这会改变已有的字典,即,这会使得该字典在其它地方也会变成修改后的字典。为了返回新字典,需要copy字典:
def removekey(d, key): r = dict(d) del r[key] return r
dict()
构造函数是浅复制,深复制见:copy
module。需要注意,对于字典删除/分配的copy,都意味着算法的时间从常量时间变成线性时间,同时也占用了线性大小的空间。对于小字典来说,这并不是问题。但当这个重复copy操作出现在大字典中时,你可能需要一个不同的数据结构,例如HAMT(见此回答)
Crystal - vote: 390
pop
会改变字典。>>> lol = {hello: gdbye} >>> lol.pop(hello) 'gdbye' >>> lol {}`
如果想要保留原来的,就copy字典后再操作。
utdemir - vote: 101
我觉得你的解决方法的确有效。但如果你希望用其它方式解决,可以试试创建一个新字典,把不需要的键排除在外即可:
>>> a {0: 'zero', 1: 'one', 2: 'two', 3: 'three'} >>> {i:a[i] for i in a if i!=0} {1: 'one', 2: 'two', 3: 'three'}`
Delete an element from a dictionary
richzilla asked:
- Is there a way to delete an item from a dictionary in Python?
如何在Python的字典中删除一个元素? - Additionally, how can I delete an item from a dictionary to return a copy (i.e., not modifying the original)?
此外,如果我希望获得一个修改后的新字典,并且原字典还是未修改的样子,应该怎么办?
- Is there a way to delete an item from a dictionary in Python?
Answers:
Greg Hewgill - vote: 2142
The
del
statement removes an element:
del
语句可以删除元素:del d[key]
Note that this mutates the existing dictionary, so the contents of the dictionary changes for anybody else who has a reference to the same instance. To return a new dictionary, make a copy of the dictionary:
但这会改变已有的字典,即,这会使得该字典在其它地方也会变成修改后的字典。为了返回新字典,需要copy字典:def removekey(d, key): r = dict(d) del r[key] return r
The
dict()
constructor makes a shallow copy. To make a deep copy, see thecopy
module.
dict()
构造函数是浅复制,深复制见:copy
module。Note that making a copy for every dict
del
/assignment/etc. means you\'re going from constant time to linear time, and also using linear space. For small dicts, this is not a problem. But if you\'re planning to make lots of copies of large dicts, you probably want a different data structure, like a HAMT (as described in this answer).
需要注意,对于字典删除/分配的copy,都意味着算法的时间从常量时间变成线性时间,同时也占用了线性大小的空间。对于小字典来说,这并不是问题。但当这个重复copy操作出现在大字典中时,你可能需要一个不同的数据结构,例如HAMT(见此回答)Crystal - vote: 390
>>> lol = {hello: gdbye} >>> lol.pop(hello) 'gdbye' >>> lol {}`
If you want to keep the original you could just copy it.
如果想要保留原来的,就copy字典后再操作。utdemir - vote: 101
I think your solution is best way to do it. But if you want another solution, you can create a new dictionary with using the keys from old dictionary without including your specified key, like this:
我觉得你的解决方法的确有效。但如果你希望用其它方式解决,可以试试创建一个新字典,把不需要的键排除在外即可:>>> a {0: 'zero', 1: 'one', 2: 'two', 3: 'three'} >>> {i:a[i] for i in a if i!=0} {1: 'one', 2: 'two', 3: 'three'}`
共有 0 条评论