译(四十五)-Python字典排序
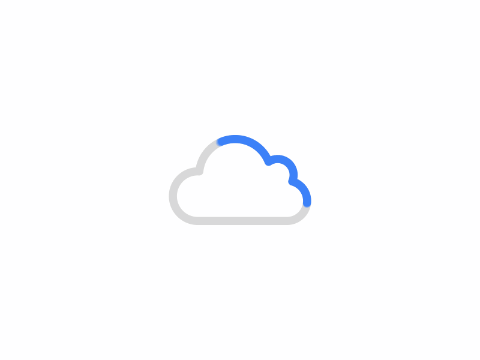
如有翻译问题欢迎评论指出,谢谢。
怎么用键排序字典?
Antony asked:
- 怎么将
{2:3, 1:89, 4:5, 3:0}
转换为{1:89, 2:3, 3:0, 4:5}
?
我找了些文章,都是用排序操作,但都返回的是元组。
- 怎么将
Answers:
NPE - vote: 1158
Python 3.7 前的标准 Python 字典都是无序的。即便键值对已经排好了,存储在字典时也无法保证顺序。
最简单的方式是使用
OrderedDict
,它可以存储插入元素的顺序。In [1]: import collections In [2]: d = {2:3, 1:89, 4:5, 3:0} In [3]: od = collections.OrderedDict(sorted(d.items())) In [4]: od Out[4]: OrderedDict([(1, 89), (2, 3), (3, 0), (4, 5)])
别介意
od
输出的方式,它会按预期那样打印。In [11]: od[1] Out[11]: 89 In [12]: od[3] Out[12]: 0 In [13]: for k, v in od.iteritems(): print k, v ....: 1 89 2 3 3 0 4 5
Python 3
对于 Python 3 的用户,需要把
iter.items()
替换为.items()
:In [13]: for k, v in od.items(): print(k, v) ....: 1 89 2 3 3 0 4 5
James - vote: 482
字典本身是无序的,如果你需要按顺序输出它们,见下面例子 :
在 Python 2.4 及以上版本:
mydict = {'carl':40, 'alan':2, 'bob':1, 'danny':3} for key in sorted(mydict): print "%s: %s" % (key, mydict[key])
输出:
alan: 2 bob: 1 carl: 40 danny: 3
Python 2.4 以下版本:
keylist = mydict.keys() keylist.sort() for key in keylist: print "%s: %s" % (key, mydict[key])
参考: http://www.saltycrane.com/blog/2007/09/how-to-sort-python-dictionary-by-keys/
Dipu - vote: 305
对于 CPython、PyPy 3.6 以及高于 3.7 版本的 Python 中,能很容易实现:
>>> d = {2:3, 1:89, 4:5, 3:0} >>> dict(sorted(d.items())) {1: 89, 2: 3, 3: 0, 4: 5}
How can I sort a dictionary by key?
Antony asked:
- What would be a nice way to go from
{2:3, 1:89, 4:5, 3:0}
to{1:89, 2:3, 3:0, 4:5}
?
I checked some posts but they all use the sorted operator that returns tuples.
怎么将{2:3, 1:89, 4:5, 3:0}
转换为{1:89, 2:3, 3:0, 4:5}
?
我找了些文章,都是用排序操作,但都返回的是元组。
- What would be a nice way to go from
Answers:
NPE - vote: 1158
Standard Python dictionaries are unordered (until Python 3.7). Even if you sorted the (key,value) pairs, you wouldn\'t be able to store them in a
dict
in a way that would preserve the ordering.
Python 3.7 前的标准 Python 字典都是无序的。即便键值对已经排好了,存储在字典时也无法保证顺序。The easiest way is to use
OrderedDict
, which remembers the order in which the elements have been inserted:
最简单的方式是使用OrderedDict
,它可以存储插入元素的顺序。In [1]: import collections In [2]: d = {2:3, 1:89, 4:5, 3:0} In [3]: od = collections.OrderedDict(sorted(d.items())) In [4]: od Out[4]: OrderedDict([(1, 89), (2, 3), (3, 0), (4, 5)])
Never mind the way
od
is printed out; it\'ll work as expected:
别介意od
输出的方式,它会按预期那样打印。In [11]: od[1] Out[11]: 89 In [12]: od[3] Out[12]: 0 In [13]: for k, v in od.iteritems(): print k, v ....: 1 89 2 3 3 0 4 5
Python 3
For Python 3 users, one needs to use the
.items()
instead of.iteritems()
:
对于 Python 3 的用户,需要把iter.items()
替换为.items()
:In [13]: for k, v in od.items(): print(k, v) ....: 1 89 2 3 3 0 4 5
James - vote: 482
Dictionaries themselves do not have ordered items as such, should you want to print them etc to some order, here are some examples:
字典本身是无序的,如果你需要按顺序输出它们,见下面例子 :In Python 2.4 and above:
在 Python 2.4 及以上版本:mydict = {'carl':40, 'alan':2, 'bob':1, 'danny':3} for key in sorted(mydict): print "%s: %s" % (key, mydict[key])
gives:
输出:alan: 2 bob: 1 carl: 40 danny: 3
(Python below 2.4:)
Python 2.4 以下版本:keylist = mydict.keys() keylist.sort() for key in keylist: print "%s: %s" % (key, mydict[key])
Source: http://www.saltycrane.com/blog/2007/09/how-to-sort-python-dictionary-by-keys/
参考: http://www.saltycrane.com/blog/2007/09/how-to-sort-python-dictionary-by-keys/Dipu - vote: 305
For CPython/PyPy 3.6, and any Python 3.7 or higher, this is easily done with:
对于 CPython、PyPy 3.6 以及高于 3.7 版本的 Python 中,能很容易实现:>>> d = {2:3, 1:89, 4:5, 3:0} >>> dict(sorted(d.items())) {1: 89, 2: 3, 3: 0, 4: 5}
共有 0 条评论