译(三十五)-Python获取类型的标准方法
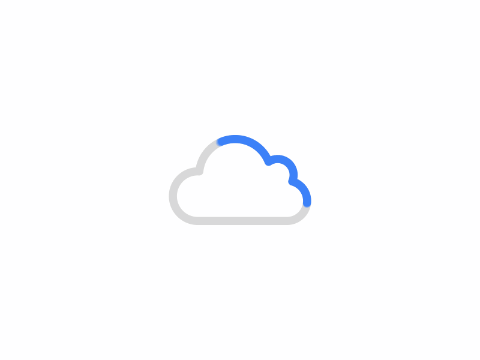
如有翻译问题欢迎评论指出,谢谢。
之前有一篇类似的译(三十一)-Python获取变量类型,这次的是另一个角度的方法和解答,更详细。
Python 获取类型的标准方法
Herge asked
- 判断对象类型的最好方式是什么?以及如何确定对象是否继承于给定的类型?
- 例如有一个对象
o
,如何确定它是不是str
?
Answers
Fredrik Johansson - vote 1828
判断
o
是否为str
或其子类,使用 isinstance (这是标准方式):if isinstance(o, str)
判断
o
是否为str
(不包括其子类):if type(o) is str
下面的语句也有效,并且在一些情况下很有用:
if issubclass(type(o), str)
见 Python 库参考的内置函数以获取更多信息。
此外,如果是用 Python 2,你需要的可能是这个:
if isinstance(o, basestring)
因为这会将 Unicode 字符串也纳入考虑(
unicode
不是str
的子类;str
与unicode
都是basestring
的子类)。而 Python 3 移除了basestring
,对于字符串(str
) 和二进制数据(bytes
)有了严格区分。此外,
isinstance
可以以类的元组作为参数。如果o
是(str, unicode)
的实例或子类 将返回True
:if isinstance(o, (str, unicode))
Dan Lenski - vote 239
最Python式的判断方法是...不要判断它。
因为 Python 鼓励鸭子类型,所以应该用
try...except
,按你希望的方式来使用这个对象的方法。所以如果你的函数是寻找一个可写的文件对象,不要去判断它是否是file
的子类,直接用它的.write()
方法即可。的确有时这些漂亮的抽象会失灵,那么
isinstance(obj, cls)
就变成你需要的,但还是得少用它。Herge - vote 65
isinstance(o, str)
将返回True
,如果o
是str
或继承于str
。仅当
o
是str
而非其子类时,type(o) is str
会返回True
。它会返回False
如果o
继承于str
。
What's the canonical way to check for type in Python
Herge asked
- What is the best way to check whether a given object is of a given type? How about checking whether the object inherits from a given type?
判断对象类型的最好方式是什么?以及如何确定对象是否继承于给定的类型? - Let's say I have an object
o
. How do I check whether it's astr
?
例如有一个对象o
,如何确定它是不是str
?
- What is the best way to check whether a given object is of a given type? How about checking whether the object inherits from a given type?
Answers
Fredrik Johansson - vote 1828
To check if
o
is an instance ofstr
or any subclass ofstr
, use isinstance (this would be the canonical way)
判断o
是否为str
或其子类,使用 isinstance (这是标准方式):if isinstance(o, str)
To check if the type of
o
is exactlystr
(exclude subclasses):
判断o
是否为str
(不包括其子类):if type(o) is str
The following also works, and can be useful in some cases:
下面的语句也有效,并且在一些情况下很有用:if issubclass(type(o), str)
See Built-in Functions in the Python Library Reference for relevant information.
见 Python 库参考的内置函数以获取更多信息。One more note in this case, if you're using Python 2, you may actually want to use:
此外,如果是用 Python 2,你需要的可能是这个:if isinstance(o, basestring)
because this will also catch Unicode strings (
unicode
is not a subclass ofstr
; bothstr
andunicode
are subclasses ofbasestring
). Note thatbasestring
no longer exists in Python 3, where there's a strict separation of strings (str
) and binary data (bytes
).
因为这会将 Unicode 字符串也纳入考虑(unicode
不是str
的子类;str
与unicode
都是basestring
的子类)。而 Python 3 移除了basestring
,对于字符串(str
) 和二进制数据(bytes
)有了严格区分。Alternatively,
isinstance
accepts a tuple of classes. This will returnTrue
ifo
is an instance of any subclass of any of(str, unicode)
:
此外,isinstance
可以以类的元组作为参数。如果o
是(str, unicode)
的实例或子类 将返回True
:if isinstance(o, (str, unicode))
Dan Lenski - vote 239
The most Pythonic way to check the type of an object is... not to check it.
最Python式的判断方法是...不要判断它。Since Python encourages Duck Typing, you should just
try...except
to use the object's methods the way you want to use them. So if your function is looking for a writable file object, don't check that it's a subclass offile
, just try to use its.write()
method!
因为 Python 鼓励鸭子类型,所以应该用try...except
,按你希望的方式来使用这个对象的方法。所以如果你的函数是寻找一个可写的文件对象,不要去判断它是否是file
的子类,直接用它的.write()
方法即可。Of course, sometimes these nice abstractions break down and
isinstance(obj, cls)
is what you need. But use sparingly.
的确有时这些漂亮的抽象会失灵,那么isinstance(obj, cls)
就变成你需要的,但还是得少用它。Herge - vote 65
isinstance(o, str)
will returnTrue
ifo
is anstr
or is of a type that inherits fromstr
.
isinstance(o, str)
将返回True
,如果o
是str
或继承于str
。type(o) is str
will returnTrue
if and only ifo
is a str. It will returnFalse
ifo
is of a type that inherits fromstr
.
仅当o
是str
而非其子类时,type(o) is str
会返回True
。它会返回False
如果o
继承于str
。
共有 0 条评论