译(五十九)-Pandas dataframe转PyTorch tensor
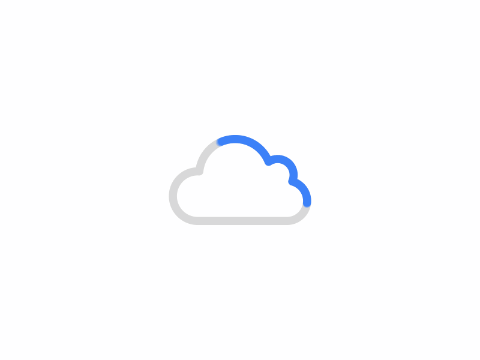
如有翻译问题欢迎评论指出,谢谢。
Pandas dataframe 转 PyTorch tensor
M. Fabio asked:
我想用 PyTorch 对 pandas dataframe
df
训练一个简单的神经网络。其中一列是
Target
,表示网络的训练目标,怎么用它作为 PyTorch 的输入?我试了下面这个但是不管用:
import pandas as pd import torch.utils.data as data_utils # target = pd.DataFrame(df['Target']) train = data_utils.TensorDataset(df, target) train_loader = data_utils.DataLoader(train, batch_size=10, shuffle=True)
Answers:
MBT - vote: 62
你好像没把确切的需求写在文中,所以我就按你的标题来回答,也就是转换 DataFrame 为 Tensor。
你的数据也没给出,那我以浮点数为例。
转换 Pandas dataframe 为 PyTorch tensor?
import pandas as pd import torch import random # # creating dummy targets (float values) targets_data = [random.random() for i in range(10)] # # creating DataFrame from targets_data targets_df = pd.DataFrame(data=targets_data) targets_df.columns = ['targets'] # # creating tensor from targets_df torch_tensor = torch.tensor(targets_df['targets'].values) # # printing out result print(torch_tensor)
输出:
tensor([ 0.5827, 0.5881, 0.1543, 0.6815, 0.9400, 0.8683, 0.4289, 0.5940, 0.6438, 0.7514], dtype=torch.float64)
PyTorch 0.4.0 环境下测试。
希望能帮到你,还有问题请追问。:)
Allen Qin - vote: 23
试试这个行不行(基于你给的代码)
train_target = torch.tensor(train['Target'].values.astype(np.float32)) train = torch.tensor(train.drop('Target', axis = 1).values.astype(np.float32)) train_tensor = data_utils.TensorDataset(train, train_target) train_loader = data_utils.DataLoader(dataset = train_tensor, batch_size = batch_size, shuffle = True)
Anh-Thi DINH - vote: 12
下面的函数可以转换任意的 Pandas dataframe/series 为 PyTorch tensor。
import pandas as pd import torch # # determine the supported device def get_device(): if torch.cuda.is_available(): device = torch.device('cuda:0') else: device = torch.device('cpu') # don't have GPU return device # # convert a df to tensor to be used in pytorch def df_to_tensor(df): device = get_device() return torch.from_numpy(df.values).float().to(device) # df_tensor = df_to_tensor(df) series_tensor = df_to_tensor(series)
Convert Pandas dataframe to PyTorch tensor?
M. Fabio asked:
I want to train a simple neural network with PyTorch on a pandas dataframe
df
.
我想用 PyTorch 对 pandas dataframedf
训练一个简单的神经网络。One of the columns is named
Target
, and it is the target variable of the network. How can I use this dataframe as input to the PyTorch network?
其中一列是Target
,表示网络的训练目标,怎么用它作为 PyTorch 的输入?I tried this, but it doesn\'t work:
我试了下面这个但是不管用:import pandas as pd import torch.utils.data as data_utils # target = pd.DataFrame(df['Target']) train = data_utils.TensorDataset(df, target) train_loader = data_utils.DataLoader(train, batch_size=10, shuffle=True)
Answers:
MBT - vote: 62
I\'m referring to the question in the title as you haven\'t really specified anything else in the text, so just converting the DataFrame into a PyTorch tensor.
你好像没把确切的需求写在文中,所以我就按你的标题来回答,也就是转换 DataFrame 为 Tensor。Without information about your data, I\'m just taking float values as example targets here.
你的数据也没给出,那我以浮点数为例。Convert Pandas dataframe to PyTorch tensor?
转换 Pandas dataframe 为 PyTorch tensor?import pandas as pd import torch import random # # creating dummy targets (float values) targets_data = [random.random() for i in range(10)] # # creating DataFrame from targets_data targets_df = pd.DataFrame(data=targets_data) targets_df.columns = ['targets'] # # creating tensor from targets_df torch_tensor = torch.tensor(targets_df['targets'].values) # # printing out result print(torch_tensor)
Output:
输出:tensor([ 0.5827, 0.5881, 0.1543, 0.6815, 0.9400, 0.8683, 0.4289, 0.5940, 0.6438, 0.7514], dtype=torch.float64)
Tested with Pytorch 0.4.0.
PyTorch 0.4.0 环境下测试。I hope this helps, if you have any further questions - just ask. 🙂
希望能帮到你,还有问题请追问。:)Allen Qin - vote: 23
Maybe try this to see if it can fix your problem(based on your sample code)?
试试这个行不行(基于你给的代码)train_target = torch.tensor(train['Target'].values.astype(np.float32)) train = torch.tensor(train.drop('Target', axis = 1).values.astype(np.float32)) train_tensor = data_utils.TensorDataset(train, train_target) train_loader = data_utils.DataLoader(dataset = train_tensor, batch_size = batch_size, shuffle = True)
Anh-Thi DINH - vote: 12
You can use below functions to convert any dataframe or pandas series to a pytorch tensor
下面的函数可以转换任意的 Pandas dataframe/series 为 PyTorch tensor。import pandas as pd import torch # # determine the supported device def get_device(): if torch.cuda.is_available(): device = torch.device('cuda:0') else: device = torch.device('cpu') # don't have GPU return device # # convert a df to tensor to be used in pytorch def df_to_tensor(df): device = get_device() return torch.from_numpy(df.values).float().to(device) # df_tensor = df_to_tensor(df) series_tensor = df_to_tensor(series)
共有 0 条评论